ToolBar を使う|Android開発
Android5.0で導入されたToolBar
の使い方です。久々に開発するとActionBar
と混乱してました。ざっくり言うと
ActionBar
はレイアウトに記載しなくても表示されるToolBar
は自分でレイアウトに追加する
ToolBar
は自分でレイアウトに追加する分、カスタマイズやActivity
毎の表示・非表示が可能になります。
ToolBar を表示する
準備
- res\values\styles.xmlに
NoActionBar
を継承したテーマを作成します
AppTheme
は任意のテーマ名です。
- AndroidManifest.xmlで
<application>
のandroid:theme
に作成したテーマ名を指定します
Activityのレイアウトファイルに記述する場合
- レイアウトルートの一番目に
android.support.v7.widget.Toolbar
を追加します
- Activityの
onCreate()
でsetSupportActionBar()
をコールし、ToolBar
をActionBar
として動作するようにします
ソースコードで動的に追加する場合
複数の画面で同じToolBar
を使用する場合などはこちらが便利です。
ToolBar
のレイアウトファイルを作成します
my_toolbar.xml
- Activityの
onCreate()
で下記を記述し、ToolBar
を追加します
LayoutInflater
はXMLからViewを作成します。第3パラメータは第2パラメータをルートにするかどうかです
- Activtyのコンテンツ部分に
ActionBar
の高さだけマージンを取ります
表示例
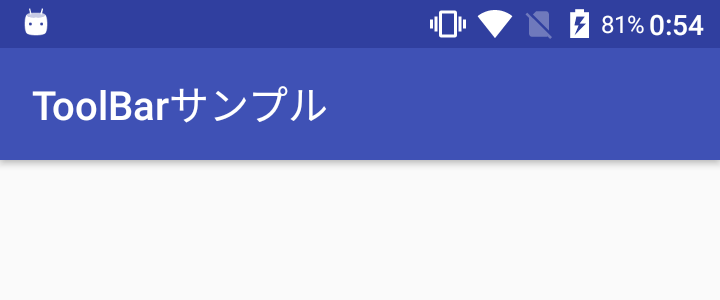
ActionBar の navigationIcon の色を変えたい場合
ActivityのonCreate()
でActionBar
設定後、下記をコールするとナビゲーションを表示できます。
この時、アイコンの色は黒系で表示されます。
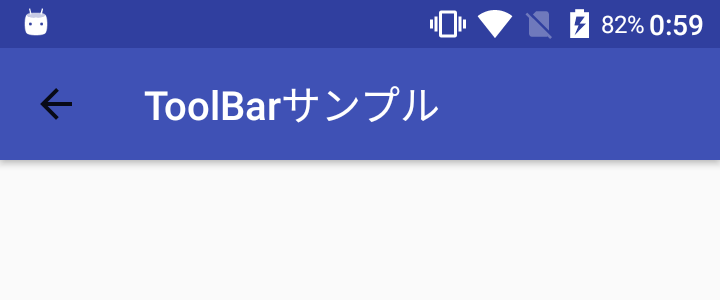
アイコンの色を変更したい場合はレイアウトファイルでapp:navigationIcon
でアイコンを指定します。
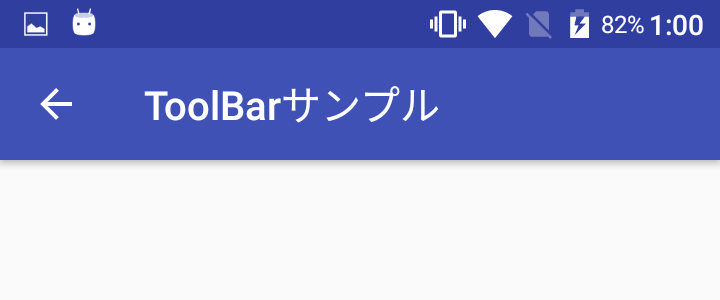
但し、この場合はsetDisplayHomeAsUpEnabled(true);
をしなくても必ずナビゲーションアイコンが表示されるようになります。共通XMLからToolBar
を生成していて、表示したくない画面がある場合はActivityのonCreate()
で下記を記述します。
ナビゲーションアイコンタップ時の処理を記述する
ActivityのonOptionsItemSelected()
をオーバーライドして処理を記述します。下記は表示中のActivityを終了するサンプルです。
super.onBackPressed();
の代わりに下記でもActivityが終了します。
メニューを表示する場合
ToolBar
にメニューを追加する方法です。
メニューを作成する
res\menu\にメニュー定義を追加します。
toolbar_menu.xml
ToolBar にメニューを追加する
ActivityでonCreateOptionsMenu()
をオーバーライドし、メニューを追加します。
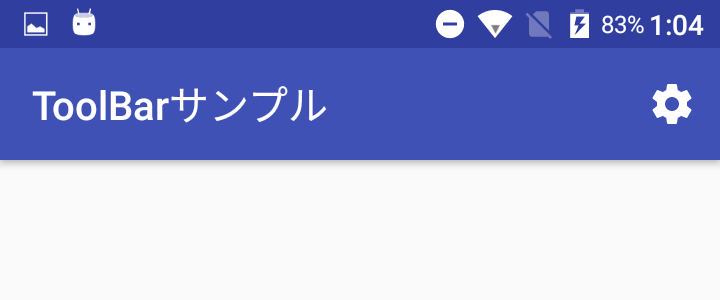
メニュータップ時の処理
ActivityのonOptionsItemSelected()
をオーバーライドして処理を記述します。