トップレベルメニューを追加する|WordPressテーマ作成
WordPressのテーマ作成でトップレベルのメニューに設定ページを追加する方法です。
add_menu_page()
を使ってトップレベルのメニューページを追加します。
下記はfunctions.php
に追加用のクラスを記述し、生成することで設定の追加を行うサンプルです。
設定画面の追加
<?php
/*
* トップレベルメニューを追加.
*/
class My_Theme_Settings_Page {
public static $option_name = 'mt_option_name';
private $options;
function __construct() {
add_action( 'admin_menu', array( $this, 'add_theme_page' ) );
add_action( 'admin_init', array( $this, 'page_init' ) );
}
/**
* 設定画面の追加.
*/
public function add_theme_page() {
add_menu_page(
'My Theme Settings', // Page title
'My Theme 設定', // Menu title
'manage_options', // Capability
'mt-admin-slug', // Menu slug
array( $this, 'create_page' )
);
}
/**
* 画面の出力.
*/
public function create_page() {
if ( !current_user_can( 'manage_options' ) ) {
wp_die( __( 'You do not have sufficient permissions to access this page.' ) );
}
// Set class property
$this->options = get_option( self::$option_name );
?>
<div class="wrap">
<h1>My Theme Settings</h1>
<form method="post" action="options.php">
<?php
// This prints out all hidden setting fields.
settings_fields( 'mt_option_group' );
do_settings_sections( 'mt-admin-slug' );
submit_button();
?>
</form>
</div>
<?php
}
/**
* 設定の追加.
*/
public function page_init() {
register_setting(
'mt_option_group', // Option group
self::$option_name, // Option name
array( $this, 'sanitize' ) // Sanitize
);
add_settings_section(
'setting_section_id', // ID
'Section 1', // Title
array( $this, 'print_section_callback' ), // Callback
'mt-admin-slug' // Page (Menu slug)
);
add_settings_section(
'setting_section_id2', // ID
'Section 2', // Title
array( $this, 'print_section_callback' ), // Callback
'mt-admin-slug' // Page (Menu slug)
);
add_settings_field(
'id_number', // ID
'Number', // Title
array( $this, 'number_callback' ), // Callback
'mt-admin-slug', // Page (Menu slug)
'setting_section_id' // Section
);
add_settings_field(
'id_title',
'Title',
array( $this, 'title_callback' ),
'mt-admin-slug',
'setting_section_id'
);
add_settings_field(
'id_number2', // ID
'Number2', // Title
array( $this, 'number2_callback' ), // Callback
'mt-admin-slug', // Page (Menu slug)
'setting_section_id2' // Section
);
}
public function sanitize( $input ) {
$new_input = array();
if ( isset( $input['id_number'] ) ) {
$new_input['id_number'] = absint( $input['id_number'] );
}
if ( isset( $input['id_title'] ) ) {
$new_input['id_title'] = sanitize_text_field( $input['id_title'] );
}
if ( isset( $input['id_number2'] ) ) {
$new_input['id_number2'] = absint( $input['id_number2'] );
}
return $new_input;
}
/**
* セクション説明.
*/
public function print_section_callback() {
print 'Enter your settings below:';
}
/**
* Get the settings option array and print one of its values.
*/
public function number_callback() {
printf(
'<input type="text" id="id_number" name="' . self::$option_name . '[id_number]" value="%s" />',
isset( $this->options['id_number'] ) ? esc_attr( $this->options['id_number']) : ''
);
}
/**
* Get the settings option array and print one of its values
*/
public function title_callback() {
printf(
'<input type="text" id="id_title" name="'. self::$option_name . '[id_title]" value="%s" />',
isset( $this->options['id_title'] ) ? esc_attr( $this->options['id_title']) : ''
);
}
/**
* Get the settings option array and print one of its values.
*/
public function number2_callback() {
printf(
'<input type="text" id="id_number2" name="' . self::$option_name . '[id_number2]" value="%s" />',
isset( $this->options['id_number2'] ) ? esc_attr( $this->options['id_number2']) : ''
);
}
}
if ( is_admin() ) {
$my_settings_page = new My_Theme_Settings_Page();
}
page_init() の処理
add_settings_field()
で設定値を定義します。パラメータにadd_settings_section()
のIDを記述して、設定画面内の表示位置を決定します。
register_setting()
で設定を登録します。
追加されたメニュー
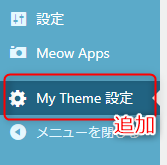
設定画面
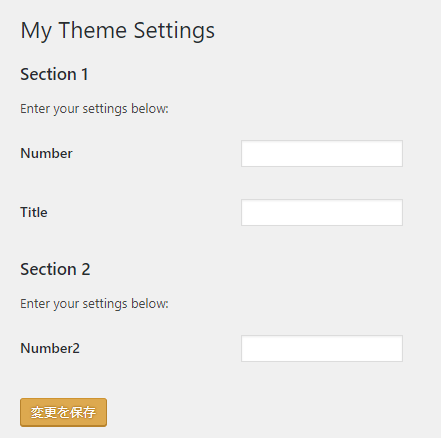
設定値の使用例
<?php $options = get_option( My_Theme_Settings_Page::$option_name ); ?>
<?php echo esc_html( $options['id_title'] );?>