サブメニューを追加する|WordPressテーマ作成
WordPressのテーマ作成でサブメニューの設定ページを追加する方法です。
基本的にはトップレベルメニューを追加すると同じで使用する関数が異なるだけです。
下記はadd_options_page()
を使って設定メニューにサブメニューページを追加するサンプルです。
サブメニュー設定画面の追加
<?php
/*
* 既存のトップメニューにサブメニューを追加する.
* 設定のサブメニューを追加する.
*/
class OGP_Settings_Page {
public static $option_name = 'options_ogp';
private $options;
function __construct() {
add_action( 'admin_menu', array( $this, 'add_submenu_page' ) );
add_action( 'admin_init', array( $this, 'page_init' ) );
}
/**
* 設定画面の追加.
*/
public function add_submenu_page() {
add_options_page(
'OGP 設定', // Page title
'OGP', // Menu title
'manage_options', // Capability
'menu-slug-options-ogp', // Menu slug
array( $this, 'create_page' ) /*, $icon_url, $position*/
);
}
/**
* 画面の出力.
*/
public function create_page() {
if ( !current_user_can( 'manage_options' ) ) {
wp_die( __( 'You do not have sufficient permissions to access this page.' ) );
}
// Set class property
$this->options = get_option( self::$option_name );
?>
<div class="wrap">
<h1>OGP 設定</h1>
<form method="post" action="options.php">
<?php
// This prints out all hidden setting fields.
settings_fields( 'options_ogp_group' );
do_settings_sections( 'menu-slug-options-ogp' );
submit_button();
?>
</form>
</div>
<?php
}
/**
* 設定の追加.
*/
public function page_init() {
register_setting(
'options_ogp_group', // Option group
self::$option_name, // Option name
array( $this, 'sanitize' ) // Sanitize
);
add_settings_section(
'options_ogp_common', // ID
'共通', // Title
null,
'menu-slug-options-ogp' // Page (Menu slug)
);
add_settings_section(
'options_ogp_facebook', // ID
'Facebook', // Title
array( $this, 'print_section_fb_callback' ), // Callback
'menu-slug-options-ogp' // Page (Menu slug)
);
add_settings_field(
'og_type', // ID
'og:type', // Title
array( $this, 'type_callback' ), // Callback
'menu-slug-options-ogp', // Page (Menu slug)
'options_ogp_common' // Section
);
add_settings_field(
'og_image', // ID
'og:image(URL)', // Title
array( $this, 'image_callback' ), // Callback
'menu-slug-options-ogp', // Page (Menu slug)
'options_ogp_common' // Section
);
add_settings_field(
'og_fb_app_id', // ID
'fb:app_id', // Title
array( $this, 'fb_app_id_callback' ), // Callback
'menu-slug-options-ogp', // Page (Menu slug)
'options_ogp_facebook' // Section
);
add_settings_field(
'og_fb_admins', // ID
'fb:admins', // Title
array( $this, 'fb_admins_callback' ), // Callback
'menu-slug-options-ogp', // Page (Menu slug)
'options_ogp_facebook' // Section
);
}
public function sanitize( $input ) {
$new_input = array();
if ( isset( $input['og_type'] ) ) {
$new_input['og_type'] = $input['og_type'];
}
if ( isset( $input['og_image'] ) ) {
$new_input['og_image'] = esc_url( $input['og_image'] );
}
if ( isset( $input['og_fb_app_id'] ) ) {
$new_input['og_fb_app_id'] = esc_attr( $input['og_fb_app_id'] );
}
if ( isset( $input['og_image'] ) ) {
$new_input['og_fb_admins'] = esc_attr( $input['og_fb_admins'] );
}
return $new_input;
}
/**
* セクション説明.
*/
function print_section_fb_callback() {
print 'fb:app_id または fb:admins';
}
/**
* Get the settings option array and print one of its values.
*/
function type_callback() {
print "\n" . '<select id="og_type" name="'. self::$option_name . '[og_type]">' . "\n";
$og_type = isset( $this->options['og_type'] ) ? $this->options['og_type'] : '';
if ( $og_type == 'blog' ) {
$selected_value = 'selected';
} else {
$selected_value = '';
}
print ' <option value="blog" label="blog" ' . $selected_value. '>blog</option>' . "\n";
if ( $og_type == "website" ) {
$selected_value = 'selected';
} else {
$selected_value = '';
}
print ' <option value="website" label="website" ' . $selected_value. '>website</option>' . "\n";
print '</select>' . "\n";
}
/**
* Get the settings option array and print one of its values.
*/
function image_callback() {
printf(
'<input type="text" id="og_image" name="' . self::$option_name . '[og_image]" value="%s" />',
isset( $this->options['og_image'] ) ? esc_url( $this->options['og_image']) : ''
);
print "\n";
}
/**
* Get the settings option array and print one of its values.
*/
function fb_app_id_callback() {
printf(
'<input type="text" id="og_fb_app_id" name="' . self::$option_name . '[og_fb_app_id]" value="%s" />',
isset( $this->options['og_fb_app_id'] ) ? esc_attr( $this->options['og_fb_app_id']) : ''
);
}
/**
* Get the settings option array and print one of its values.
*/
function fb_admins_callback() {
printf(
'<input type="text" id="og_fb_admins" name="' . self::$option_name . '[og_fb_admins]" value="%s" />',
isset( $this->options['og_fb_admins'] ) ? esc_attr( $this->options['og_fb_admins']) : ''
);
print "\n";
}
}
if ( is_admin() ) {
$ogp_settings_page = new OGP_Settings_Page();
}
追加されたメニュー
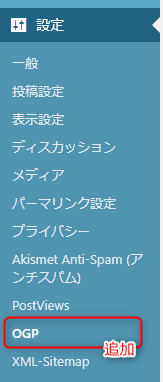
設定画面
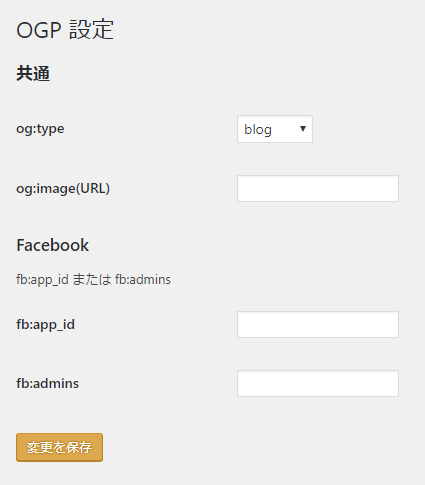
設定値の使用例
<?php $options_ogp = get_option( OGP_Settings_Page::$option_name ); ?>
<meta property="og:type" content="<?php echo $options_ogp['og_type']; ?>" />
<meta property="og:image" content="<?php echo $options_ogp['og_image']; ?>" />
<meta property="fb:app_id" content="<?php echo $options_ogp['og_fb_app_id']; ?>" />