オリジナルのウィジェットを追加する|WordPressテーマ作成
外観>ウィジェット>利用できるウィジェットにカスタムウィジェットを追加する方法です。
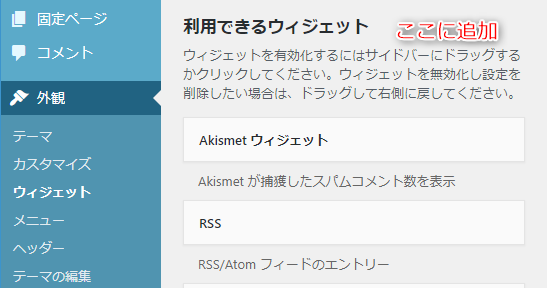
ウィジェットの作成
functions.php
にWP_Widget
を継承したclass
を定義します
このクラスがウィジェットとして追加されます- コンストラクタでID、表示名、概要を指定します
表示イメージ
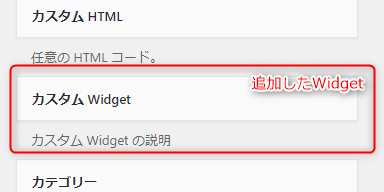
widget
メソッドでWidget実行時の出力を記述します
第1引数はウィジェットの引数です。register_sidebar
の値が取得できます
第2引数はデータベースに保存されているウィジェットの値です
register_sidebar
については、
独自サイドバー(サイドバーウィジェット)の追加方法|WordPressテーマ作成
を参照ください。
form
メソッドで管理画面でのフォーム出力を記述します
第1引数はデータベースに保存されているウィジェットの値です
フォームに指定するid属性、name属性はそれぞれ$this->get_field_id( 'キー名' )
、$this->get_field_name( 'キー名' )
で指定しなければなりません。
表示イメージ
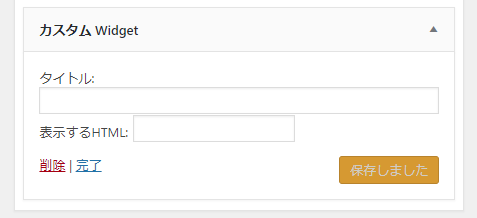
update
メソッドで保存処理を記述します
第1引数は新しくフォームに入力された値
第2引数はデータベースに保存されている前回の値です
戻り値として返した配列が実際に保存されます
利用できるウィジェットの追加
functions.php
でwidgets_init
フックを使い、register_widget()
で作成したウィジェットを追加します。
サンプル
設定例
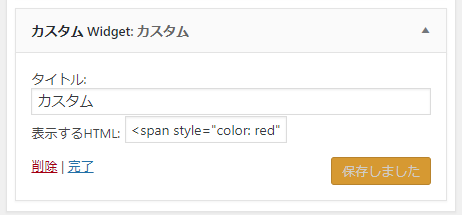
出力例
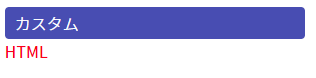